Maximizing your WordPress site with API Integration
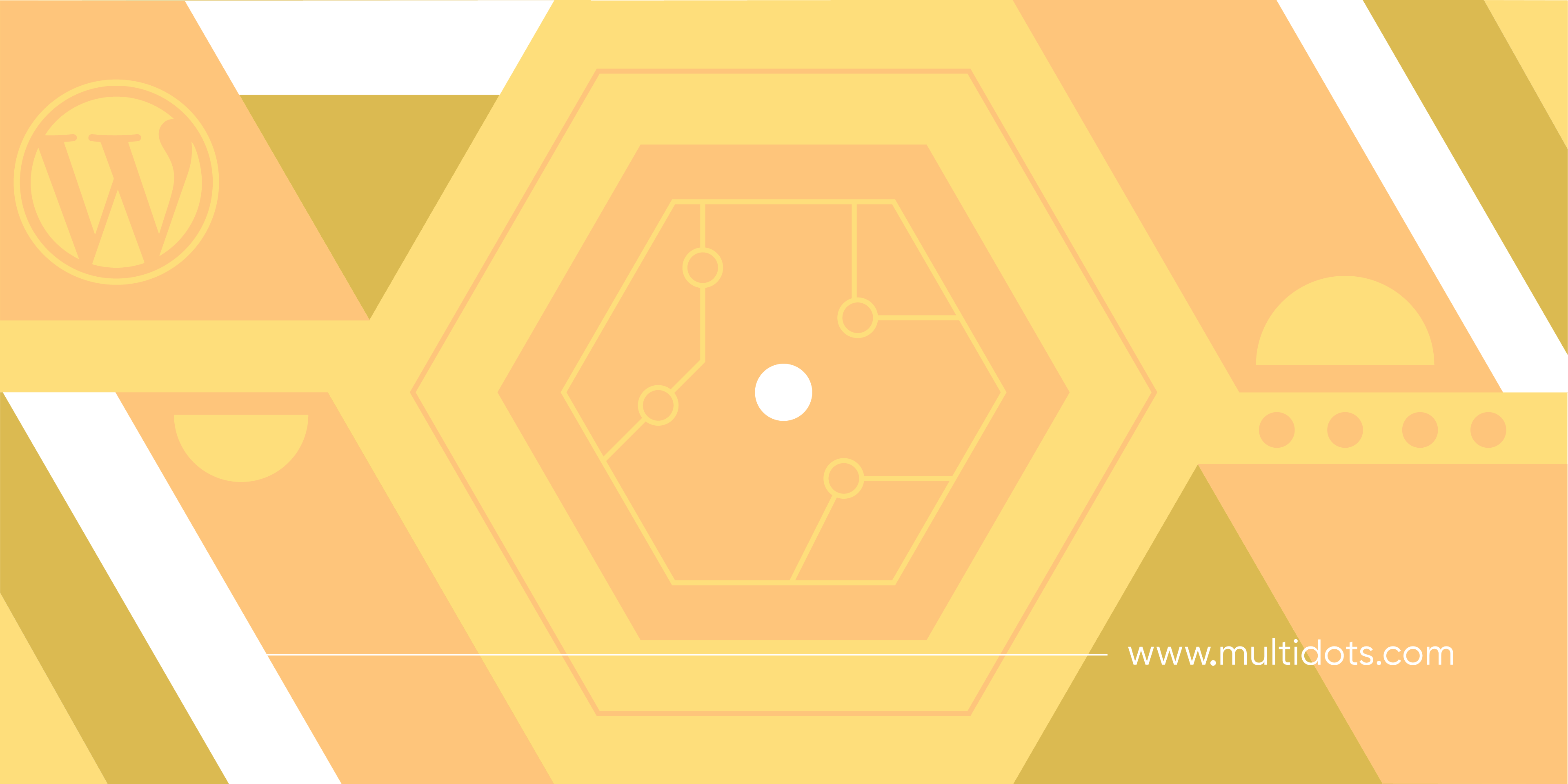
Table of Contents
APIs (Application Programming Interfaces) are important for creating dynamic and interactive WordPress websites. They are a set of rules and protocols that enable programs to talk to each other, allowing developers to fetch and display real-time data, integrate third-party services, and create custom features without altering the core WordPress code.
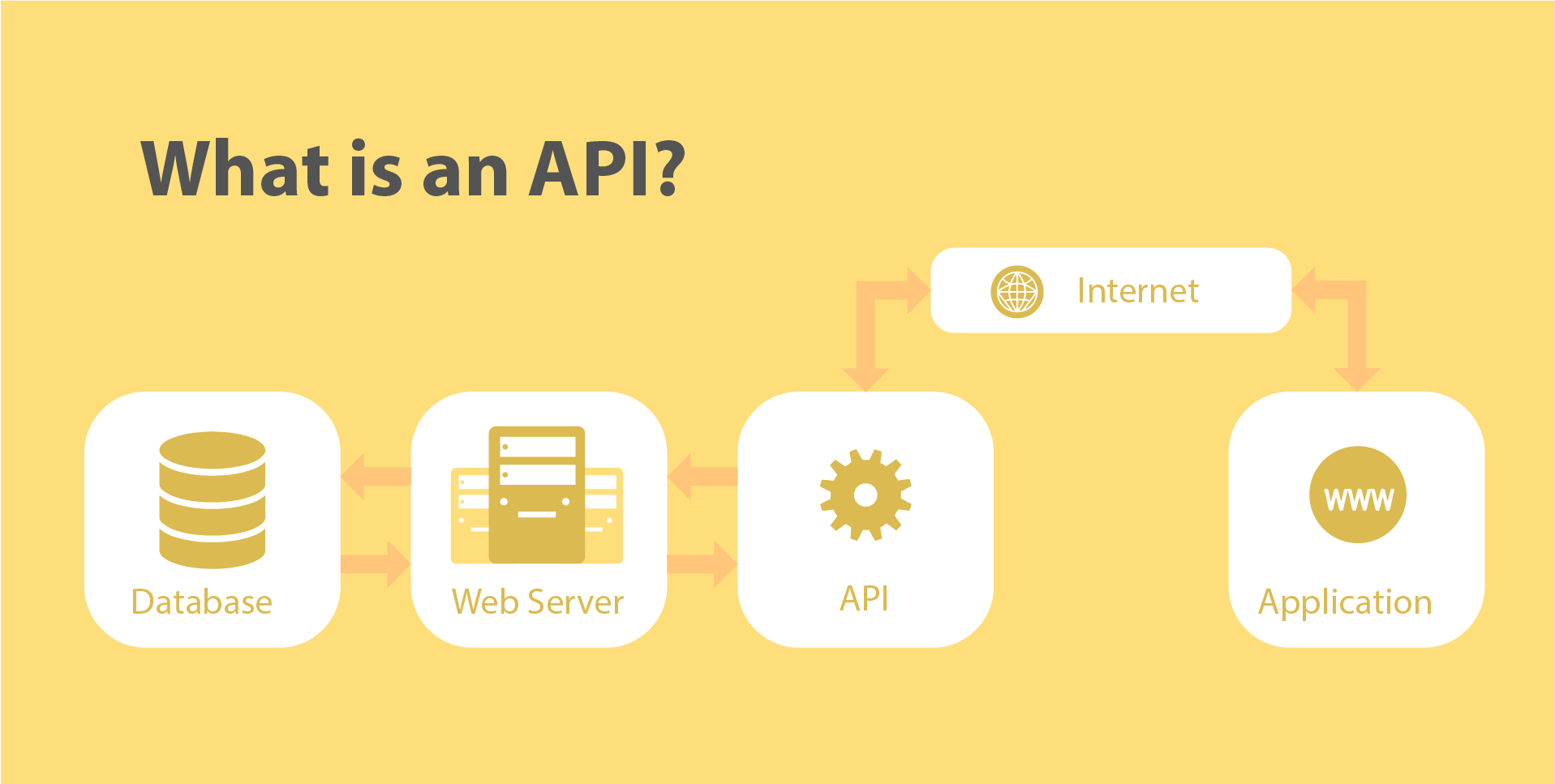
The key API concepts include:
- Interface: provides a way for applications to interact with each other.
- Protocol: defines how requests and responses should be formatted and processed.
- Abstraction: hides the complexity of underlying implementations, offering simpler ways to access functionalities.
- Reusability: which promotes code reuse by enabling developers to leverage existing functions or data.
The WordPress REST API is a specific type of web API that WordPress provides for interacting with its platform programmatically. Some of its practical uses entail displaying social media feeds, integrating payment gateways, and synchronizing data with external CRMs.
While understanding and handling APIs can be complicated, the benefits – such as enhanced site functionality, improved user experience, and tailored custom solutions – are well worth the effort.
An Overview of WordPress REST API
The WordPress REST API expands how you can use WordPress beyond its traditional web interface. It opens up possibilities for accessing WordPress data and functionalities from outside the WordPress environment, enabling the creation of more dynamic and interactive applications. You can perform various actions, such as retrieving or updating content, from external applications or websites without directly interacting with the WordPress admin panel.
REST (Representational State Transfer), a set of rules and protocols, plays a big part in making this possible and effortless. Let’s unpack the key principles of REST in order to understand its crucial role in the WordPress REST API:
- Stateless Communication: Communication between the client and server is stateless, meaning each request from the client to the server must contain all the information needed to understand and process the request. The server doesn't store any session information about the client.
- Client-Server Architecture: The client and server are separate entities. The client is responsible for the user interface and user experience, while the server handles the backend logic and data storage. This separation allows for independent evolution and scalability of both components.
- Resource-Based: In REST, everything is considered a resource. This allows developers to access and manipulate WordPress data (like posts, pages, users, etc.) using standard HTTP methods (GET, POST, PUT, DELETE).
- Representation of Resources: Resources are typically represented in JSON (JavaScript Object Notation) format, which is a lightweight data-interchange format that is easy to read and write for humans and easy to parse and generate for machines.
These principles ensure efficient, scalable, and easy-to-maintain communication between different parts of a WordPress site and external applications. That’s why developers rely on the WordPress REST API to interact programmatically with WordPress data to build custom front-ends or integrate WordPress with other services.
Components of WordPress REST API
Endpoints: Endpoints are the access points for interacting with the WordPress REST API. They are specific URLs that allow applications to access and manipulate WordPress data. Common endpoints include /wp-json/wp/v2/posts
for posts, /wp-json/wp/v2/pages
for pages, and /wp-json/wp/v2/users
for users.
Custom Endpoints: Developers can create custom endpoints to extend the REST API's functionality, tailoring it to specific needs and enhancing site capabilities.
Plugins like WPGetAPI simplify managing these components, especially for users who may not be comfortable with coding, making API integration more accessible.
Routes: Routes map URLs to specific endpoints. They define how API requests are structured and directed to the correct endpoints. For example, the route /wp-json/wp/v2/posts
corresponds to the posts endpoint, enabling access to WordPress posts.
Requests: The REST API supports various types of requests:
- GET: Retrieve data e.g., fetch posts or pages.
- POST: Create new data e.g., add a new post.
- PUT: Update existing data e.g., modify a post.
- DELETE: Remove data e.g., delete a post.
Responses: API responses include the requested data, usually formatted in JSON. The responses also contain status codes indicating the success or failure of the request, such as 200 OK for success or 404 Not Found for errors.
Authentication: Authentication allows for secure interactions with the API. Methods include cookie authentication, basic authentication, and OAuth. Each method has its own use case, ensuring that only authorized users can access or modify data.
The Role of REST API in Building a WordPress Website
Dynamic Content
The REST API means you can fetch and display dynamic content, significantly enhancing the user experience. It enables real-time updates without requiring page reloads, making sites more interactive and responsive. For example, a news site can use the REST API to automatically update headlines and articles as they are published.
Headless WordPress
Headless WordPress refers to the decoupling of the frontend from the backend, using the REST API to serve content to various front-end technologies. This approach offers several benefits:
- Improved Performance: By separating the content management system from the presentation layer, performance can be optimized for each independently.
- Flexibility: Developers can use any frontend framework or technology they prefer, such as React.
- Scalability: The ability to handle high traffic and complex operations more efficiently.
Custom Applications
The REST API allows developers to create custom applications tailored to specific needs. For example:
- Custom Dashboards: Personalized admin panels that offer unique insights and controls.
- Content Management Tools: Applications that streamline content creation, editing, and management.
- E-commerce Integrations: Custom shopping experiences and payment gateways.
Improved Workflows
Content creators and developers benefit from the REST API as it simplifies workflows. It allows for the synchronization of content across various platforms, minimizing the necessity for manual updates and maintaining consistency.
Enhanced Security
The REST API boosts security through granting authority over entry to particular endpoints. Various authentication methods like cookie authentication, basic authentication, and OAuth guarantee safe data transfer and protect against unauthorized entry.
How to Integrate APIs into WordPress
Theme and Plugin Integration
Theme Integration
Integrating APIs directly into your WordPress theme involves adding API calls within theme files, such as your child theme’s functions.php. For instance, you might use the wp_remote_get()
function to make HTTP GET requests in WordPress to fetch data. Here’s a basic example:
function fetch_api_data() { $response = wp_remote_get('https://api.example.com/data'); if (is_array($response) && !is_wp_error($response)) { $body = json_decode($response['body'], true); return $body; } return null; }
The wp_remote_get()
function, which is part of the WordPress HTTP API, sends a GET request to the specified URL ('https://api.example.com/data'). The result of this request is stored in the $response variable.
if (is_array($response) && !is_wp_error($response))
checks if the $response is an array and does not contain any errors. If this check passes, this line decodes the JSON response body into a PHP array. The json_decode()
function converts the JSON string into a PHP array (since it has a true parameter).
To display the data you fetched with this code, you need to add additional code to process and output the data within a WordPress template or a specific hook like so:
get_header(); ?> <div class="content"> <?php // Fetch the data from the API $posts = fetch_api_data(); // Check if data was returned if ($posts) { // Loop through the posts and display them foreach ($posts as $post) { ?> <div class="post"> <h2><?php echo esc_html($post['title']); ?></h2> <p><?php echo esc_html($post['content']); ?></p> </div> <?php } } else { echo '<p>No posts available.</p>'; } ?> </div> <?php get_footer(); ?>
Don’t forget, when integrating APIs, it's important to enqueue scripts and styles correctly using wp_enqueue_script()
and wp_enqueue_style()
to ensure they load properly and avoid conflicts.
Plugin Integration
Plugins provide a convenient method for incorporating APIs into websites without the need to modify theme files. They ensure easy updates and maintenance, making them ideal for users who want to avoid dealing with theme code. Well-known plugins for API integration are WPGetAPI and Custom API for WP.
Using plugins for API integration offers several benefits:
- Ease of Updates: Plugins can be updated independently of the theme.
- Maintenance: Separates custom functionality from theme files, reducing the risk of losing changes during theme updates.
- Child Themes: Employ child themes to protect customizations from being overwritten during theme updates.
Proper documentation and code commenting are essential for maintainability, ensuring that any modifications or customizations can be easily understood and managed in the future.
Boosting Functionality with WPGetAPI Plugin
With WPGetAPI, you can display API data using shortcodes or template tags, making it easy to integrate dynamic content into your site. The plugin also allows for extensions with actions, tokens, and dynamic variables, offering flexibility for more complex API interactions.
Error handling and logging are also needed for troubleshooting API issues, and WPGetAPI includes features to manage these effectively. Along with this, caching API responses can significantly improve performance by reducing the need for repeated API calls.
WPGetAPI is compatible with plugins like WooCommerce, Elementor, and Advanced Custom Fields (ACF), enhancing site functionality through integration with these popular tools. This compatibility allows for the creation of more dynamic and interactive WordPress sites, tailored to specific needs.
A Step-by-Step Guide to Using WPGetAPI
Tools and Knowledge Database
- Basic Understanding of REST APIs: Familiarize yourself with basic concepts such as endpoints, routes, and HTTP request methods (GET, POST, etc.).
- WordPress Setup: Ensure you have a WordPress site up and running.
- Postman is a great tool for testing API requests before integrating them into your site.
Here’s how to get started:
Step #1: Install and Configure WPGetAPI
- Go to your WordPress dashboard.
- Navigate to Plugins → Add New.
- Search for WPGetAPI and click Install Now.
- Activate the plugin.
Step #2: Identify the External API You Want to Integrate and Sign Up for an API Key
For example, if you want to fetch and display current weather data for a specific city, you can use weatherapi.com.
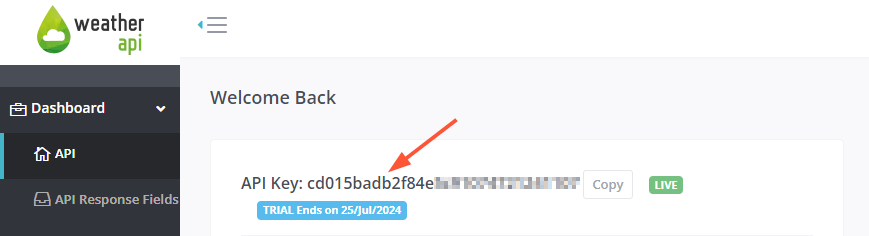
Step #3: Configure the API on WPGetAPI
- Once activated, go to WPGetAPI → Setup.
- Enter your API Name (e.g., "MyWeatherAPP"), Unique ID, and Base URL (e.g., https://api.openweathermap.org/data/2.5/), then save your changes.
- In the next section, enter the endpoint URL, request method (GET, POST, etc.), result response (JSON), and any required parameters.
- Click on the new API tab that was created and fill in the fields to set up the API endpoint you want to use:
- Endpoint Name: A name to identify this specific endpoint.
- Unique ID: A unique lowercase ID for the endpoint.
- Endpoint: The path to the specific endpoint from the API docs.
- Method: The HTTP method (GET, POST, PUT, DELETE).
- Authorization: Select the authorization type if required.
- Fill in any required Query Parameters, Headers, and Body Parameters.
- Click save.
Step #4: Test the Endpoint
- After saving the endpoint, the Test Endpoint button will become active. Click it to call the API and return the data. Alternatively, you can navigate to the endpoint URL using a tool like Postman.
- Check the Data Output section to verify you are getting the expected data.
Step #5: Displaying API Data Using WPGetAPI Shortcodes and Template Tags
- Using Shortcodes: WPGetAPI provides shortcodes to display API data in your posts or pages:
[wpgetapi_endpoint endpoint_id="1"]
- Using Template Tags: Alternatively, you can use the function provided by the WPGetAPI plugin in your child theme's template files, like so:
$api_data = wpgetapi_endpoint('your_api_id', 'your_endpoint_id'); wpgetapi_pp($api_data);
Advanced Techniques for API Integration
Creating Custom Routes and Endpoints
Here’s a simple overview of a custom REST API endpoint for fetching posts that other applications, services, or frontend JavaScript can call:
Step #1: Register Custom Route
Add the following code snippet in your child theme’s functions.php file:
add_action('rest_api_init', function () { register_rest_route('custom/v1', '/data', [ 'methods' => 'GET', 'callback' => 'get_custom_data', 'permission_callback' => '__return_true', // This can be replaced with a custom permission callback function ]); });
This registers a route at wp-json/custom/v1/data
with the GET method.
Step #2: Create a Callback Function to Handle the GET Request and Fetch Posts
Write the logic for retrieving the posts and preparing the response. Here’s an example:
/** * Callback function to handle the GET request and fetch posts. * * @param WP_REST_Request $request Full data about the request. * @return WP_REST_Response|WP_Error */ function get_custom_data(WP_REST_Request $request) { // Define the query arguments $args = array( 'post_type' => 'post', // Change this if you want to fetch custom post types 'post_status' => 'publish', 'posts_per_page' => 10, // Number of posts to fetch ); // Execute the query $query = new WP_Query($args); // Prepare the response data $posts = array(); if ($query->have_posts()) { while ($query->have_posts()) { $query->the_post(); $posts[] = array( 'id' => get_the_ID(), 'title' => get_the_title(), 'content' => get_the_content(), 'excerpt' => get_the_excerpt(), 'link' => get_permalink(), ); } wp_reset_postdata(); } // Return the response return new WP_REST_Response($posts, 200); }
Step #3: Define the Permission Callback
Specify which users you want to allow to access the API. Here’s an example of allowing only the logged-in users to access the endpoint:
* @return bool function custom_permission_callback() { return is_user_logged_in(); }
Step #4: Test Your Custom Endpoint
You can test your custom endpoint by navigating to the following URL in your browser or using a tool like Postman:
https://your-wordpress-site.com/wp-json/custom/v1/data
Improving Performance with Caching Strategies
Caching enhances performance by storing frequently accessed data and reducing server load. There are different types of caching:
- Object Caching: Stores database query results to reduce repeated queries.
- Page Caching: Saves rendered pages to serve them quickly to users.
- Transient Caching: Uses WordPress transients for temporary caching of API responses.
Some best practices for doing this are:
- Secure Cached Data: Encrypt sensitive cached data.
- Regular Invalidation: Ensure cached data is updated periodically to reflect the latest information.
Dealing with Common Challenges in API Integration
Security
Securing API integrations is essential to protect sensitive data and prevent unauthorized access. Several methods and best practices can ensure the security of your API integrations:
- OAuth and API Tokens: Use OAuth for secure token-based authentication, allowing users to authorize applications to interact with their data without sharing credentials. API tokens also provide a secure way to authenticate requests.
- Data Encryption: Encrypt data both in transit (using HTTPS) and at rest to safeguard sensitive information from interception and unauthorized access.
- Regular Updates: Keep APIs and their dependencies updated to mitigate vulnerabilities. Security patches and updates address known issues and protect against emerging threats.
Managing Complexity and Compatibility
Integrating multiple APIs from different providers can present challenges, such as compatibility and data consistency issues. Here are some strategies to manage these complexities:
- Unified Interface Tools: Use tools like Postman and Swagger to manage and test APIs. These platforms offer a consistent interface for handling multiple APIs, simplifying development and debugging processes.
- Data Consistency: Make sure data consistency across different APIs by implementing synchronization mechanisms. Regularly check for data discrepancies and resolve them promptly.
- Error Handling and Exception Management: Implement error-handling mechanisms to maintain application stability. Detect errors in real time using logging and monitoring solutions, and provide clear, actionable error messages to users. Fallback mechanisms can ensure business continuity even when some API calls fail.
Error Handling and Exception Management
Effective error handling is essential to maintain the stability of your application. Here are some techniques:
- Logging and Monitoring: Use logging tools to capture and monitor errors in real time. Solutions like Sentry or Loggly can help detect and diagnose issues quickly.
- Clear Error Messages: Provide users with clear and actionable error messages to help them understand and resolve issues.
- Fallback Mechanisms: Implement fallback mechanisms to handle API failures gracefully. This could include using cached data or alternative APIs to ensure continuous operation.
Performance Optimization
Slow APIs can negatively impact user experience and site performance. Here are some strategies to optimize API performance:
- Caching Strategies: Implement caching to reduce API load and improve response times. Use techniques like object caching, page caching, and transient caching.
- Optimized Database Queries: Ensure database queries are optimized to enhance performance. Efficient queries can reduce the time required to fetch data from the database.
- Content Delivery Networks (CDNs): Use CDNs to improve API performance and reduce latency by serving content from servers closer to the user.
Mastering API Integration in WordPress with Multidots
API integration offers significant benefits for WordPress websites, including dynamic content updates, integration with external services, and enhanced functionality. Throughout this guide, we've shown you the API integration process using the WPGetAPI plugin, which simplifies the task and requires minimal coding knowledge.
However, even with these tools, API integration can be challenging, especially for large or complex sites. For these scenarios, professional assistance ensures secure and efficient implementation.
Multidots specializes in building WordPress sites for enterprise-level clients. With our expertise, we can handle your API integrations, ensuring optimal performance and security.
Ready to enhance your WordPress site with API integrations? Get in touch with Multidots today!
Book Your Free CMS Consultation Now!
Schedule a consultation call and discuss your WordPress requirements.
(or Contact Us)